Configuration
The app configuration is the place where everything happens, all features, functions and contents are implemented here. To get started, you will make a few simple changes to the basic application and add an action to it.
This chapter therefore deals with:
This will give you a basic understanding of the configuration and interactions of layers and actions.
Starting position
Let's first get a brief overview of what the basic single page app you have created looks like.
- Open the folder containing your app in Visual Studio Code.
- Navigate to
layout > startView.json
.
This is where you will put most of your code for now.
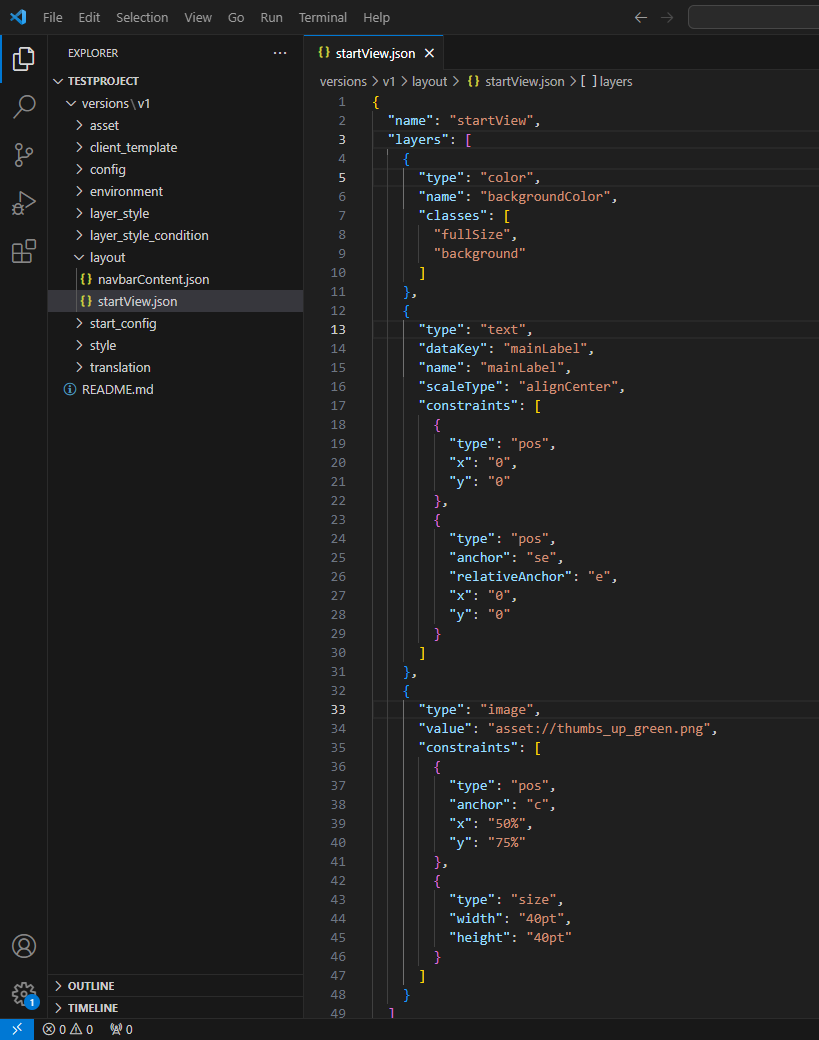
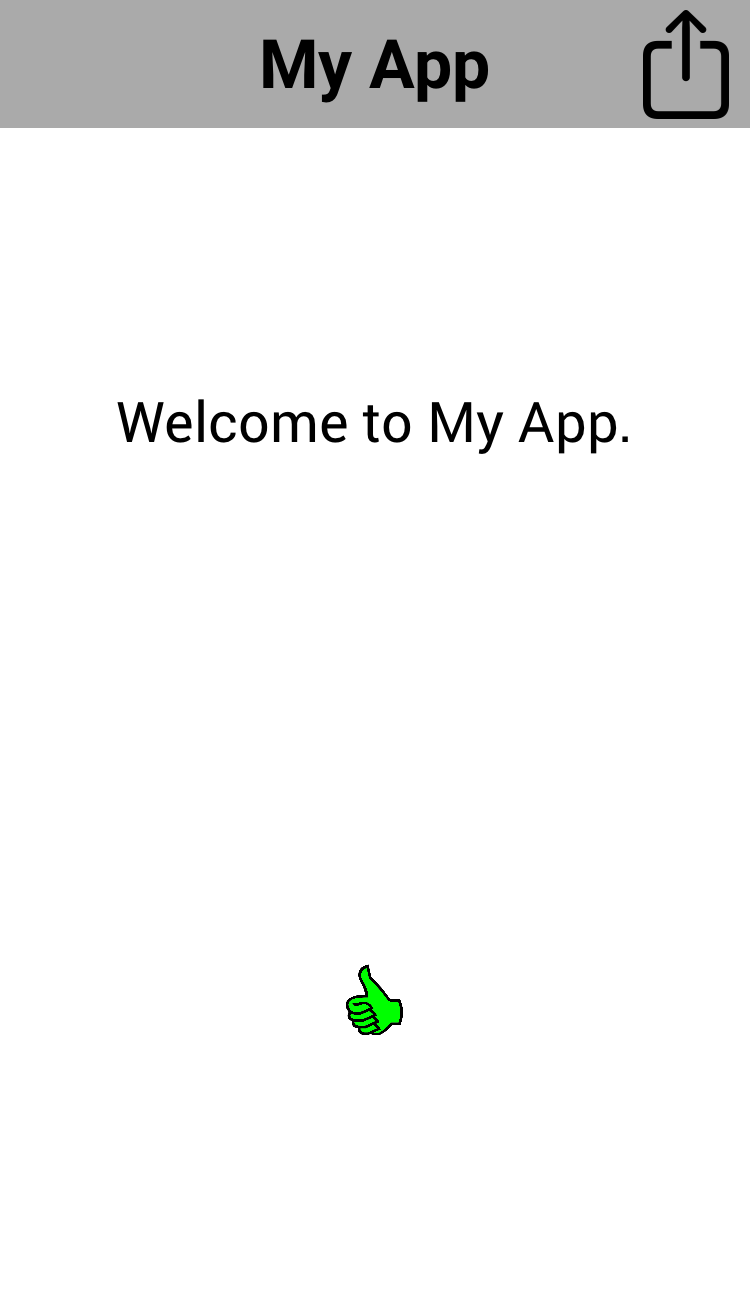
There are already three different layers in the startView.json
file that correspond to the different elements you see in the app
- One layer of the
type: color
drawing a background - One layer of the
type: text
displaying the text "Welcome to My App." - One layer of the
type: image
displaying the green thumbs up icon.
You will change the values of the color and text layer and add an action to the text layer.
Changing the background color
The color layer is responsible for drawing the background of your app. In this case it gets its value from the background
class.
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"background"
]
}
Following these instructions you will be able to change the value of the color layer and thus define a different background color for your app.
- Navigate to
layer-style > background.json
{
"name": ".background",
"content": {
"value": "#ffffff"
}
}
The value is set to #ffffff
which is the hex color code for white.
- Set the value to
#1c8fc2
or another hex color code of your choice. - Save your changes and update your test app.
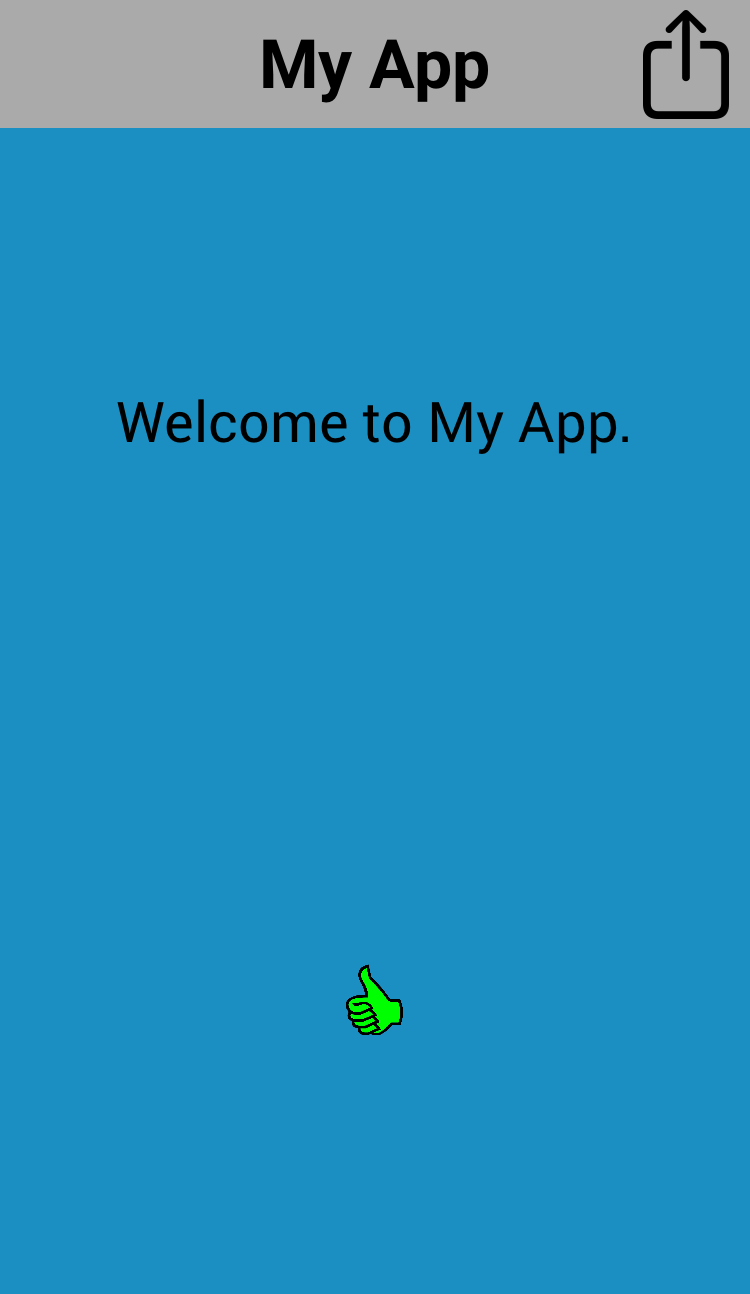
The background color of your app has now changed.
Changing the main text
The text layer is responsible for displaying the text which, which is "Welcome to My App." by default. This is not a static value, but serves as a placeholder for translations. In this case, implemented by the dataKey: mainLabel
. This allows the app to display the text in the system language if corresponding translations are available.
{
"type": "text",
"dataKey": "mainLabel",
"name": "mainLabel",
"scaleType": "alignCenter",
"constraints": [
{
"type": "pos",
"x": "0",
"y": "0"
},
{
"type": "pos",
"x": "0",
"y": "0",
"anchor": "se",
"relativeAnchor": "se"
}
]
}
Following these instructions you will be able to change the value of the text layer and define a new text to be displayed in your app.
- Navigate to
translation > default.json
or the corresponding file for your language.
{
"name": "default",
"content": {
"title": "My App",
"mainLabel": "Welcome to My App."
}
}
The value for mainLabel
, which is the dataKey
specified in the color layer, is set to "Welcome to My App."
- Set the value for
mainLabel
to "Hello World." or a different text of your choice. - Save your changes and update your test app.
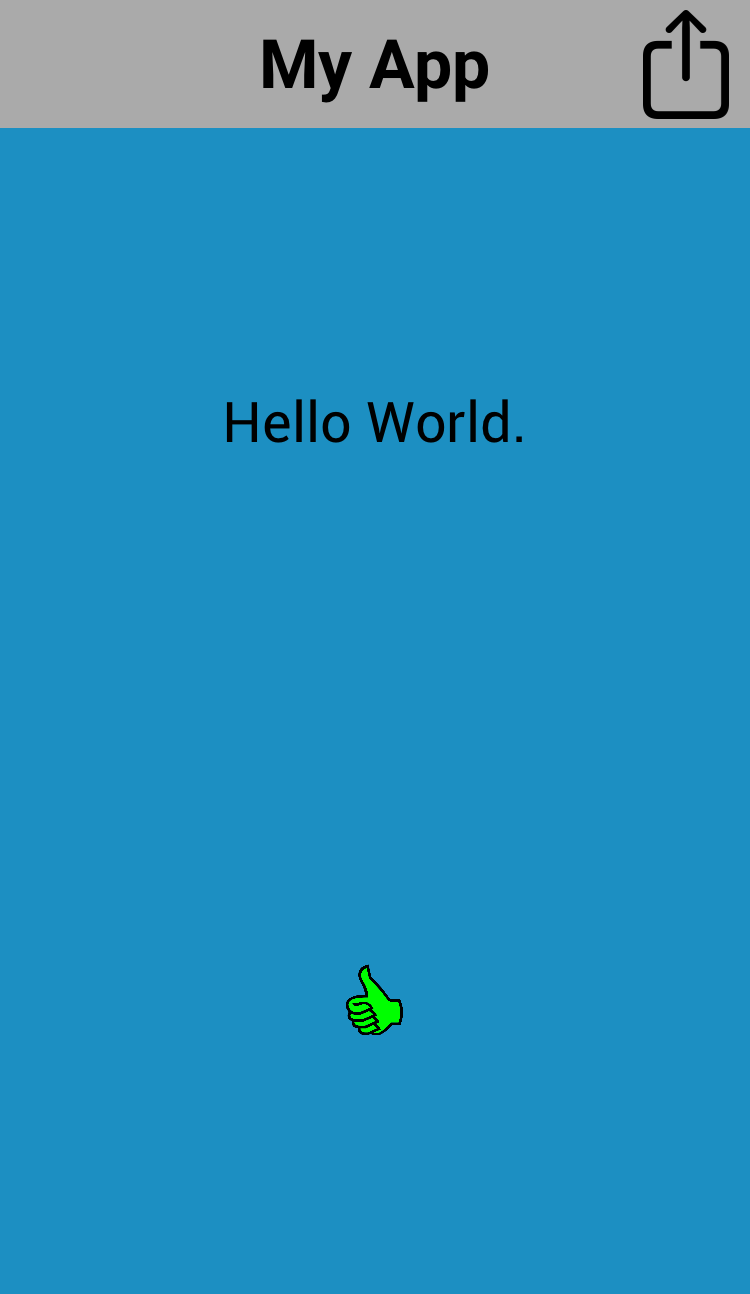
The main text of your app has now changed.
Adding an action to the text layer
Actions are added to specific layers and can perform a multitude of different operations. The showMessage
action used here displays a message after the corresponding layer has been tapped.
Following these instructions you will be able to add an action to the text Layer in layout > startView.json
, which will displays a Message after tapping the layer.
{
"constraints": [
...
],
"actions": [
]
}
- Add a new
actions
array below theconstraints
array of the text layer.
{
"actions": [
{
}
]
}
- Add a new object within the
actions
array.
{
"actions": [
{
"type": "showMessage"
}
]
}
- Add an action of the
type: showMessage
.
{
"actions": [
{
"type": "showMessage",
"params": {
}
}
]
}
- Add
params
.
{
"actions": [
{
"type": "showMessage",
"params": {
"text": "$lang(showMessageText)$"
}
}
]
}
- Add a new field
text
toparams
. - Set the value for
text
to$lang(showMessageText)$
This serves as a placeholder for translations, similar to the dataKey in the section on changing the main text.
Now you need to provide a value for showMessageText
in the translations files.
- Navigate to
translation > default.json
or the corresponding file for your language.
"showMessageText": "Hello there."
- Add a new field
showMessageText
. - Set the value for
showMessageText
to "Hello there." or a different text of your choice. - Save your changes and update your test app.
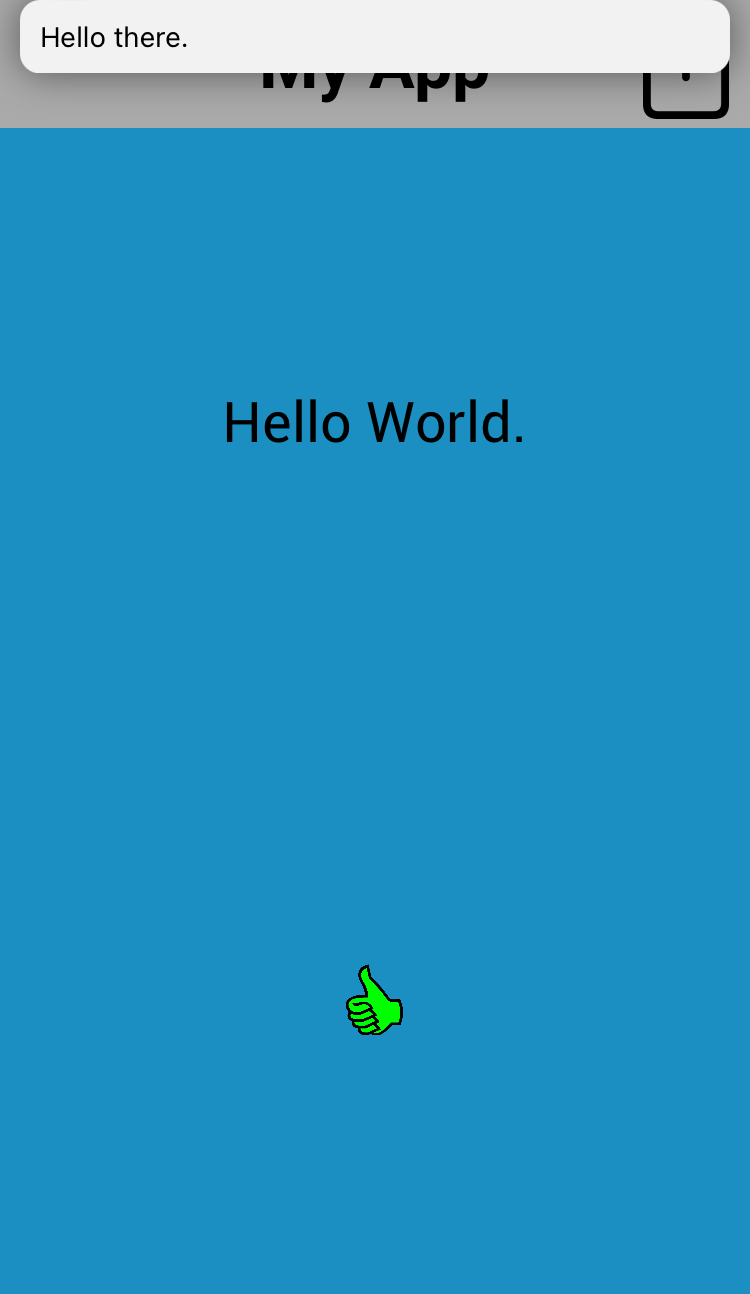
If you tap on the main text, your message should appear.
The showMessage action is now attached to the text layer.
You have now taken your first steps in mos. and are on the way to create your own apps. If you want to find out about possible next steps, take a lok at the How-tos.