Creating a Navbar
A navbar can be a useful tool fpor navigation within your app. The layout of the navbar must be defined in the style file, which means that different layout for the navbar require different styles.
In this tutorial two navigation bar layouts are created. One that displays a logo and offers no functions, and one that takes the user back to the previous view.
You can skip straight to the copyable code of this tutorial or follow the step by step instructions.
The following mos. components are used in this tutorial. If needed, you can refer to the corresponding wiki pages:
- backInHistory action
- color layer
- image layer
- layer_style
- showView action
- start_config
- style
- text layer
Step by step
Following these instructions you will be able to create a navbar for your app.
To display a navbar, the style file must first be edited to refer to the layout of the navbar and set its height.
{
"name": "default",
"content": {
"content": {
"default": {
"navbarLayout": {
"default": "navbar"
},
"navbarHeights":{
"default": "56pt"
}
}
}
}
}
- Go to the
default.json
file in the/style
directory. - Add a
navbarLayout
field and set thedefault
value tonavbar
. - Add a
navbarHeights
field and set thedefault
value to56pt
or your prefered height.
The next step is to create the corresponding layout file for the navbar. It contains the content (i.e. layers and actions) of the navbar.
{
"name": "navbar",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"navbarBackground"
]
}
]
}
- Create a new file
navbar.json
in the/layout
directory. - Add a
name
field and set its value tonavbar
. - Add a
layers
array. - Add a
color
layer to thelayers
array. This will set the background color of the navbar.
The color
layer can have the class fullSize
, as it is restricted by the dimensions of the navbar.
The value for the color
layer is specified in the navbarBackground
class so it can also be used in other navbar layouts.
{
"name": "navbar",
"layers": [
{
"type": "color",
...
},
{
"type": "image",
"value": "asset://mos_logo_text.png",
"scaleType": "scaleAspectFit",
"constraints": [
{
"type": "pos",
"anchor": "c",
"x": "50%",
"y": "50%"
},
{
"type": "size",
"height": "50pt",
"width": "200pt"
}
]
}
]
}
- Add an
image
layer to thelayers
array, e.g. to display a logo in the navbar.
You can add any number of layers to customize the navbar according to your preferences.
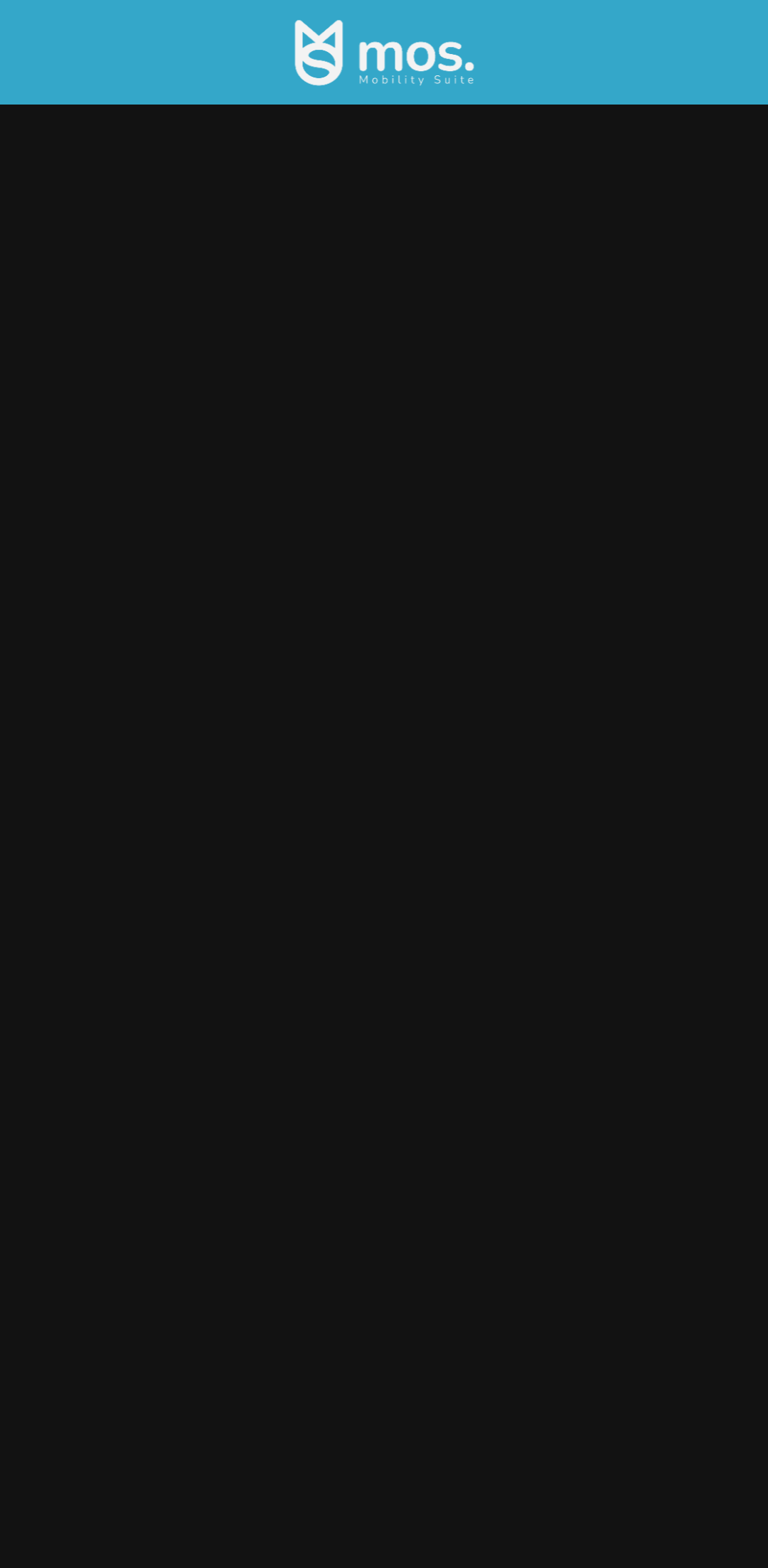
Add any content you want to the default view.
{
"name": "startView",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"background"
]
},
{
"type": "text",
"name": "viewLabel",
"value": "startView",
"scaleType": "alignCenter",
"classes": [
"text"
],
"constraints": [
{
"type": "pos",
"anchor": "c",
"x": "50%",
"y": "50%"
},
{
"type": "size",
"height": "50pt",
"width": "200pt"
}
]
}
]
}
- Go to the
startView.json
file in the/layout
directory. - Add a
text
layer to thelayers
array. - Set the value of the
text
layer tostartView
as an indicator of the current view.
Add a button to the layout to change the view later on.
{
"name": "startView",
"layers": [
{
"type": "color",
...
},
{
"type": "text",
...
},
{
"type": "container",
"name": "showViewButton",
"classes": [
"buttonContainer"
],
"children": [
{
"type": "color",
"value": "#34a7c9",
"classes": [
"fullSize"
]
},
{
"type": "text",
"value": "showView",
"scaleType": "alignCenter",
"classes": [
"fullSize"
]
}
]
}
]
}
- Add a
container
layer to thelayers
array to create a button. - Add a
children
array to thecontainer
layer. - Add a
color
and atext
layer to thechildren
array to build the button.
{
"name": "startView",
"layers": [
{
"type": "color",
...
},
{
"type": "text",
"name": "viewLabel",
...
},
{
"type": "container",
"name": "showViewButton",
"classes": [
...
],
"children": [
{
"type": "color",
...
},
{
"type": "text",
...
}
],
"actions": [
{
"type": "showView",
"params": {
"layout": "additionalView",
"namedStyle": "style_navbarBack"
}
}
]
}
]
}
- Add an
actions
array to thecontainer
layer. - Add a
showView
action to theactions
array - Add the params
layout
with the valueadditionalView
andnamedStyle
with the valuestyle_navbarBack
to theshowView
action.
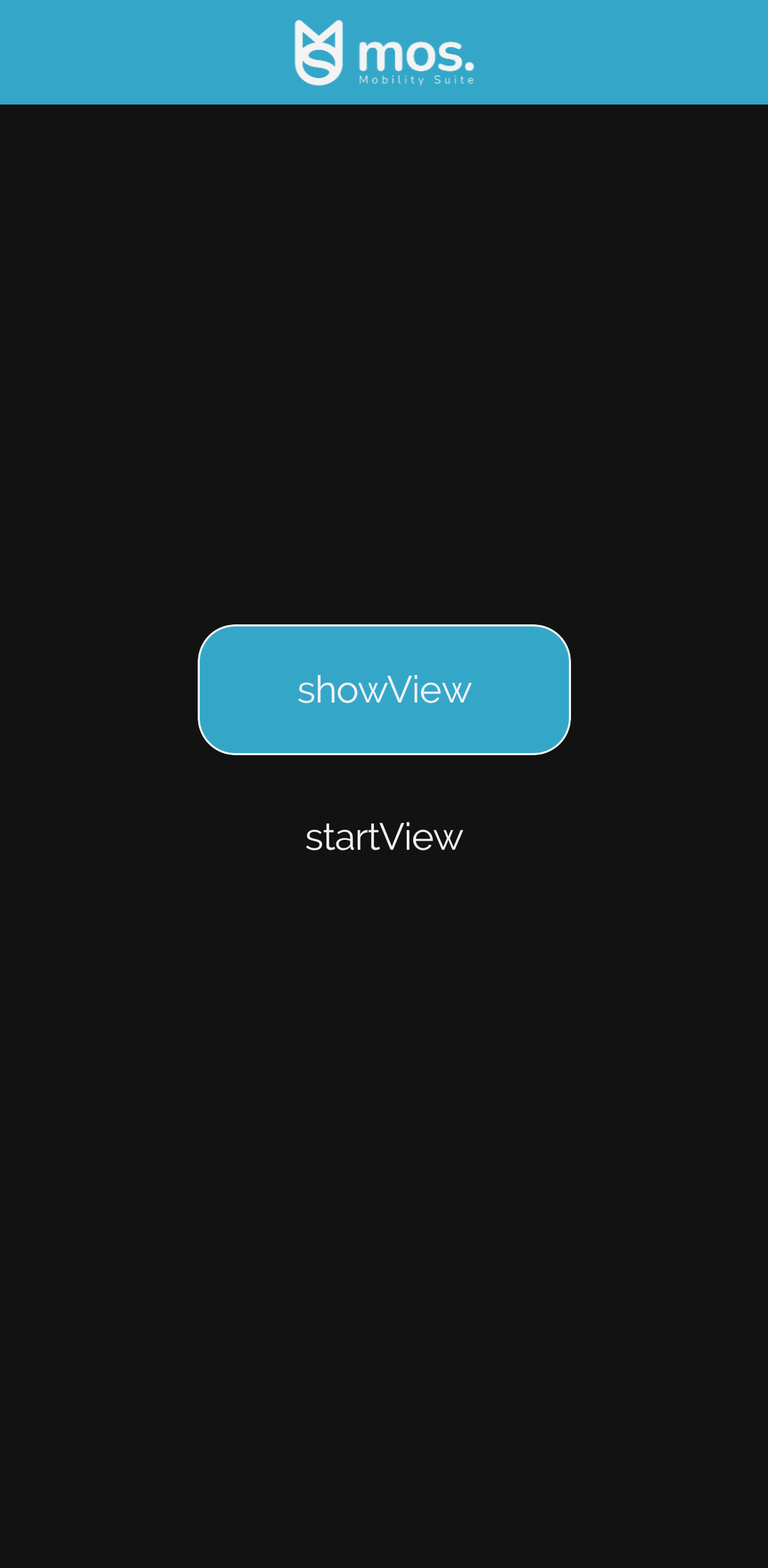
Create another layout file for the additional view to which the showView action on the button refers.
{
"name": "additionalView",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"background"
]
},
{
"type": "text",
"name": "viewLabel",
"value": "additionalView",
"scaleType": "alignCenter",
"classes": [
"text"
],
"constraints": [
{
"type": "pos",
"anchor": "c",
"x": "50%",
"y": "50%"
},
{
"type": "size",
"height": "50pt",
"width": "200pt"
}
]
}
]
}
- Create a new file
additionalView.json
in the/layout
directory. - Add a
name
field and set its value toadditionalView
. - Add a
layers
array. - Add a
color
layer to thelayers
array to set a background color. - Add a
text
layer to thelayers
array to display the current view as in thestartView.json
layout.
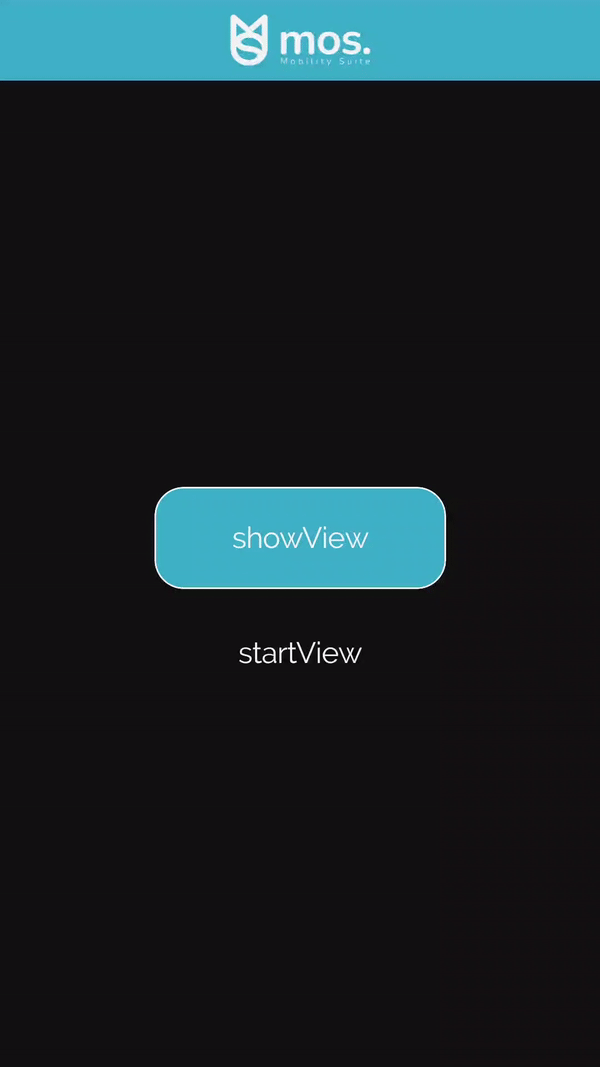
Create the style file, which is also referenced in the showView action on the button.
{
"name": "style_navbarBack",
"content": {
"content": {
"default": {
"navbarLayout": {
"default": "navbarBack"
},
"navbarHeights": {
"default": "56pt"
}
}
}
}
}
- Create a new file
style_navbarBack.json
in the/style
directory.
The structure of this file is the same as that of the default
file in the /style
directory with some values changed.
- Add a
name
field and set its value tostyle_navbarBack
. - Add a
navbarLayout
field and set thedefault
value tonavbarBack
. - Add a
navbarHeights
field and set thedefault
value to56pt
or your prefered height.
Create the new navbar layout referenced in the style just created that contains a backInHistory action for the anvigation.
{
"name": "navbarBack",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"navbarBackground"
]
}
]
}
- Create a new file
navbarBack.json
in the/layout
directory. - Add a
name
field and set its value tonavbarBack
. - Add a
layers
array. - Add a
color
layer to thelayers
array to set a background color for the navbar.
{
"name": "navbarBack",
"layers": [
{
"type": "color",
...
},
{
"type": "container",
"constraints": [
{
"type": "pos",
"anchor": "nw",
"x": "3pt",
"y": "3pt"
},
{
"type": "size",
"height": "50pt",
"width": "100pt"
}
],
"children": [
]
}
]
}
- Add a
container
layer to thelayers
array. - Add a
children
array to thecontainer
layer.
{
"name": "navbarBack",
"layers": [
{
"type": "color",
...
},
{
"type": "container",
"constraints": [
...
],
"children": [
{
"type": "text",
"name": "back",
"font": "FontAwesome",
"value": "fa-chevron-left",
"fontSize": "24pt",
"scaleType": "alignLeft",
"constraints": [
{
"type": "pos",
"anchor": "nw",
"x": "5pt",
"y": 0
},
{
"type": "size",
"height": "50pt"
}
]
}
]
}
]
}
- Add a
text
layer to thechildren
array. - Add a
font
field and set its value toFontAwesome
. - Add a
value
field and set its value tofa-chevron-left
. - Set the
constraints
to position thetext
layer on the left in the navbar.
{
"name": "navbarBack",
"layers": [
{
"type": "color",
...
},
{
"type": "container",
"constraints": [
...
],
"children": [
{
"type": "text",
...
},
{
"type": "text",
"font": "Raleway-Regular",
"value": "Back",
"fontSize": "20pt",
"scaleType": "alignLeft",
"constraints": [
{
"type": "pos",
"anchor": "nw",
"relativeAnchor": "ne",
"relativeTo": "back",
"x": "10pt",
"y": 0
},
{
"type": "size",
"height": "50pt"
}
]
}
]
}
]
}
- Add another
text
layer to thechildren
array. - Add a
value
field and set its value toBack
. - Set the
constraints
to position thetext
layer to the right of the othertext
layer.
{
"name": "navbarBack",
"layers": [
{
"type": "color",
...
},
{
"type": "container",
"constraints": [
...
],
"children": [
...
],
"actions": [
{
"type": "backInHistory"
}
]
}
]
}
- Add an
actions
array to thecontainer
layer. - Add a
backInHistory
action to theactions
array.
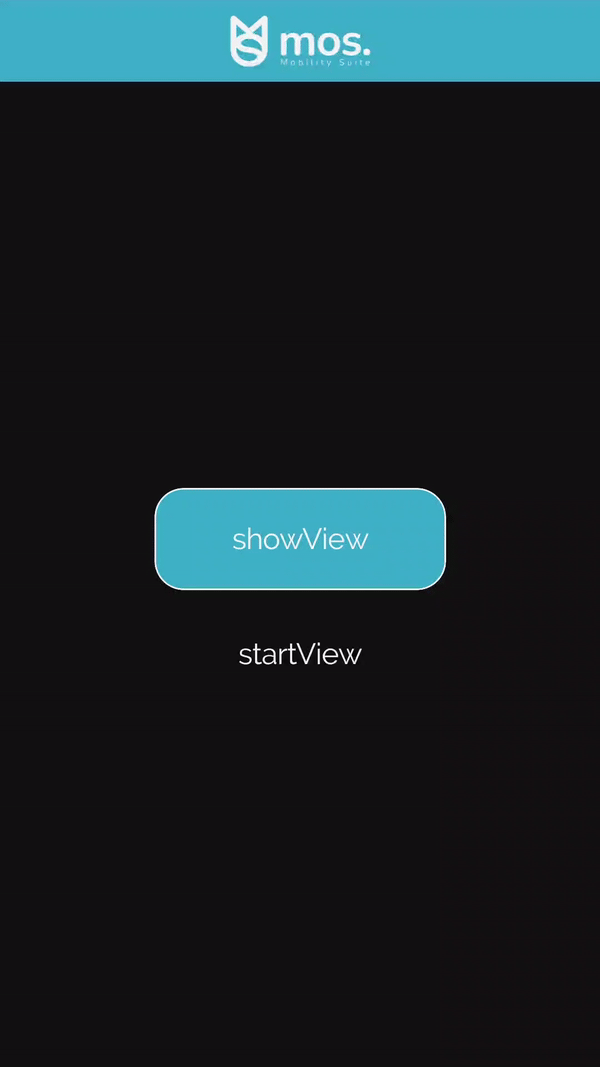
A simple navbar has been created.
Copyable code
{
"name": "default",
"content": {
"content": {
"default": {
"navbarLayout": {
"default": "navbar"
},
"navbarHeights":{
"default": "56pt"
}
}
}
}
}
{
"name": "navbar",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"navbarBackground"
]
},
{
"type": "image",
"value": "asset://mos_logo_text.png",
"scaleType": "scaleAspectFit",
"constraints": [
{
"type": "pos",
"anchor": "c",
"x": "50%",
"y": "50%"
},
{
"type": "size",
"height": "50pt",
"width": "200pt"
}
]
}
]
}
{
"name": "startView",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"background"
]
},
{
"type": "text",
"name": "viewLabel",
"value": "startView",
"scaleType": "alignCenter",
"classes": [
"text"
],
"constraints": [
{
"type": "pos",
"anchor": "c",
"x": "50%",
"y": "50%"
},
{
"type": "size",
"height": "50pt",
"width": "200pt"
}
]
},
{
"type": "container",
"name": "showViewButton",
"classes": [
"buttonContainer"
],
"children": [
{
"type": "color",
"value": "#34a7c9",
"classes": [
"fullSize"
]
},
{
"type": "text",
"value": "showView",
"scaleType": "alignCenter",
"classes": [
"fullSize"
]
}
],
"actions": [
{
"type": "showView",
"params": {
"layout": "additionalView",
"namedStyle": "style_navbarBack"
}
}
]
}
]
}
{
"name": "additionalView",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"background"
]
},
{
"type": "text",
"name": "viewLabel",
"value": "additionalView",
"scaleType": "alignCenter",
"classes": [
"text"
],
"constraints": [
{
"type": "pos",
"anchor": "c",
"x": "50%",
"y": "50%"
},
{
"type": "size",
"height": "50pt",
"width": "200pt"
}
]
}
]
}
{
"name": "additionalView",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"background"
]
},
{
"type": "text",
"name": "viewLabel",
"value": "additionalView",
"scaleType": "alignCenter",
"classes": [
"text"
],
"constraints": [
{
"type": "pos",
"anchor": "c",
"x": "50%",
"y": "50%"
},
{
"type": "size",
"height": "50pt",
"width": "200pt"
}
]
}
]
}
{
"name": "style_navbarBack",
"content": {
"content": {
"default": {
"navbarLayout": {
"default": "navbarBack"
},
"navbarHeights": {
"default": "56pt"
}
}
}
}
}
{
"name": "navbarBack",
"layers": [
{
"type": "color",
"name": "backgroundColor",
"classes": [
"fullSize",
"navbarBackground"
]
},
{
"type": "container",
"constraints": [
{
"type": "pos",
"anchor": "nw",
"x": "3pt",
"y": "3pt"
},
{
"type": "size",
"height": "50pt",
"width": "100pt"
}
],
"children": [
{
"type": "text",
"name": "back",
"font": "FontAwesome",
"value": "fa-chevron-left",
"fontSize": "24pt",
"scaleType": "alignLeft",
"constraints": [
{
"type": "pos",
"anchor": "nw",
"x": "5pt",
"y": 0
},
{
"type": "size",
"height": "50pt"
}
]
},
{
"type": "text",
"font": "Raleway-Regular",
"value": "Back",
"fontSize": "20pt",
"scaleType": "alignLeft",
"constraints": [
{
"type": "pos",
"anchor": "nw",
"relativeAnchor": "ne",
"relativeTo": "back",
"x": "10pt",
"y": 0
},
{
"type": "size",
"height": "50pt"
}
]
}
],
"actions": [
{
"type": "backInHistory"
}
]
}
]
}
{
"name": ".background",
"content": {
"value": "#121212"
}
}
{
"name": ".buttonContainer",
"content": {
"borderColor": "#f3f3f3",
"borderWidth": "1pt",
"borderRadius": "20pt",
"constraints": [
{
"type": "pos",
"anchor": "c",
"x": "50%",
"y": "40%"
},
{
"type": "size",
"width": "200pt",
"height": "70pt"
}
]
}
}
{
"name": ".fullSize",
"content": {
"constraints": [
{
"type": "pos",
"x": "0",
"y": "0"
},
{
"type": "pos",
"x": "0",
"y": "0",
"anchor": "se",
"relativeAnchor": "se"
}
]
}
}
{
"name": ".navbarBackground",
"content": {
"value": "#34a7c9"
}
}
{
"name": "text",
"content": {
"fontSize": "20pt",
"contentType": "plain",
"fontColor": "#F3F3F3",
"font": "Raleway-Regular"
}
}